1 год назад
Подскажите пожалуйста. Долбаный код не работает на Цэ++
1234567891011121314151617181920212223242526272829303132333435363738394041424344454647484950515253545556575859606162
//tv.h — клаÑÑÑ‹ Тѵ и Remote
#ifndef TV_H_
#define TV_H_
//-------------------------------------------------------------------------------------------------
class Tv
{
public:
friend class Remote; // Remote имеет доÑтуп к закрытой чаÑти Тѵ
enum { Off, On };
enum { MinVal, MaxVal = 20 };
enum { Antenna, Cable };
enum { TV, DVD };
Tv(int s = Off, int mc = 125) : state(s), volume(5),
maxchannel(mc), channel(2), mode(Cable), input(TV) {}
void onoff() { state = (state == On) ? Off : On; }
bool ison() const { return state == On; }
bool volup();
bool voldown();
void chanup();
void chandown();
void set_mode() { mode = (mode == Antenna) ? Cable : Antenna; }
void set_input() { input = (input == TV) ? DVD : TV; }
void settings() const; // display all settings
void swcont();
private:
int state; // On или Off
int volume; // диÑкретные уровни громкоÑти
int maxchannel; // макÑимальное количеÑтво каналов
int channel; // текущие наÑтройки канала
int mode; // Ñфирное или кабельное телевидение
int input; // TV или DVD
};
//-------------------------------------------------------------------------------------------------
class Remote
{
private:
int mode; // управление TV или DVD
int control;
public:
friend class Tv;
enum { normal, interactive };
void showcontrolinfo() const { if (control == normal ) std::cout << "normal" << std::endl; else std::cout << "interactive" << std::endl;};
Remote(int m = Tv::TV) : mode(m), control(normal) {}
bool volup(Tv & t) { return t.volup(); }
bool voldown(Tv & t) { return t.voldown(); }
void onoff(Tv & t) { t.onoff(); }
void chanup(Tv & t) { t.chanup(); }
void chandown(Tv & t) { t.chandown(); }
void set_chan(Tv & t, int c) { t.channel = c; }
void set_mode(Tv & t) { t.set_mode(); }
void set_input(Tv & t) { t.set_input(); }
};
//-------------------------------------------------------------------------------------------------
#endif // TV_H_
inline void Tv::swcont()
{
if (mode) control = (control == Remote::normal) ? Remote::interactive : Remote::normal;
else std::cout << "TV is off" << std::endl;
}
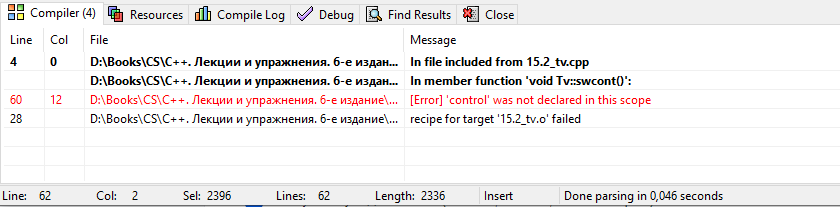
Короче объект надо в функцию посылать. тогда не понял урока с друзьями. Я думал для этого друзья и нужны чтобы иметь доступ к секретам
Только авторизированные пользователи могут оставлять свои ответы
Дата
Популярность
в Tv нет члена control, он в Remote
Тебе ж написали, что символ control не объявлен.
Я не в курсе, которая строка у тебя 60, но видимо, речь идёт о методе Tv::swcont(). В зоне его видимости нет символа control.
Выше было сказано.
12345678910111213141516171819202122232425262728293031323334353637383940414243444546474849505152535455565758596061626364656667686970717273747576
#ifndef TV_H_
#define TV_H_
#include <iostream>
class Remote;
class Tv
{
public:
friend class Remote;
enum { Off, On };
enum { MinVal, MaxVal = 20 };
enum { Antenna, Cable };
enum { TV, DVD };
Tv(int s = Off, int mc = 125)
: state(s), volume(5), maxchannel(mc), channel(2), mode(Cable), input(TV)
{}
void onoff() { state = (state == On) ? Off : On; }
bool ison() const { return state == On; }
bool volup();
bool voldown();
void chanup();
void chandown();
void set_mode() { mode = (mode == Antenna) ? Cable : Antenna; }
void set_input() { input = (input == TV) ? DVD : TV; }
void settings() const;
private:
int state;
int volume;
int maxchannel;
int channel;
int mode;
int input;
};
class Remote
{
private:
int mode;
int control;
public:
friend class Tv;
enum { normal, interactive };
Remote(int m = Tv::TV) : mode(m), control(normal) {}
bool volup(Tv& t) { return t.volup(); }
bool voldown(Tv& t) { return t.voldown(); }
void onoff(Tv& t) { t.onoff(); }
void chanup(Tv& t) { t.chanup(); }
void chandown(Tv& t) { t.chandown(); }
void set_chan(Tv& t, int c) { t.channel = c; }
void set_mode(Tv& t) { t.set_mode(); }
void set_input(Tv& t) { t.set_input(); }
void showcontrolinfo() const
{
if (control == normal)
std::cout << "normal" << std::endl;
else
std::cout << "interactive" << std::endl;
}
void swcont(Tv& t)
{
if (t.mode)
t.control = (t.control == normal) ? interactive : normal;
else
std::cout << "TV is off" << std::endl;
}
};
#endif // TV_H_