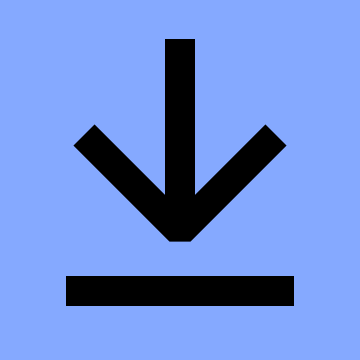
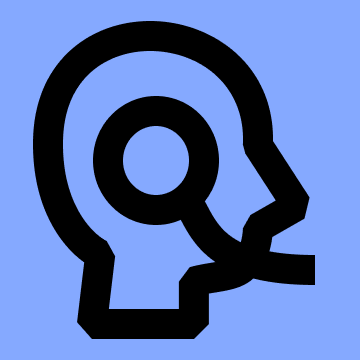
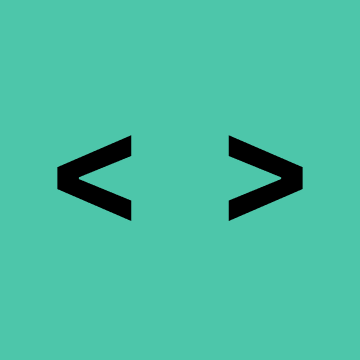
Можете кто нибудь запустить мой код c#
using System;
using System.Drawing;
using System.Windows .Forms;
namespace furrysad
{
// Это ваш класс формы
public partial class Form1 : Form
{
private Timer timer;
private float hue = 0f;
private Label label;
public Form1()
{
// Инициализация формы
this.Text = "HackerCom";
this.Size = new Size(800, 600);
this.FormBorderStyle = FormBorderStyle.None; // Без рамки
this.StartPosition = FormStartPosition.CenterScreen; // Центр экрана
this.BackColor = Color.Black ; // Черный фон
// Создание и настройка Label
label = new Label();
label.Text = "HACKERCOM";
label.Font = new Font("Arial", 48, FontStyle.Bold);
label.ForeColor = Color.White;
label.AutoSize = true;
label.Location = new Point(this.ClientSize.Width / 2 - label.Width / 2, this.ClientSize.Height / 2 - label.Height / 2);
label.TextAlign = ContentAlignment.MiddleCenter;
this.Controls.Add(label);
// Настройка таймера
timer = new Timer();
timer.Interval = 30; // Интервал обновления
timer.Tick += Timer_Tick;
timer.Start();
}
private void Timer_Tick(object sender, EventArgs e)
{
// Обновление фона с радужным эффектом
hue += 0.005f;
if (hue > 1) hue = 0;
this.BackColor = ColorFromHSV(hue, 1, 1); // Радужный цвет фона
// Сияние текста
label.ForeColor = Color.FromArgb(255,
(int)(Math.Sin(Environment.TickCount * 0.01) * 127 + 128), 255, 255);
// Центровка текста, если окно изменилось
label.Location = new Point(this.ClientSize.Width / 2 - label.Width / 2,
this.ClientSize.Height / 2 - label.Height / 2);
}
// Метод для получения цвета из HSV
public static Color ColorFromHSV(float hue, float saturation, float value)
{
int h = (int)(hue * 360);
int s = (int)(saturation * 255);
int v = (int)(value * 255);
int hi = (int)(h / 60) % 6;
float f = (h / 60f) - (int)(h / 60);
int p = (int)(v * (1 - s / 255f));
int q = (int)(v * (1 - f * s / 255f));
int t = (int)(v * (1 - (1 - f) * s / 255f));
switch (hi)
{
case 0: return Color.FromArgb(v, t, p);
case 1: return Color.FromArgb(q, v, p);
case 2: return Color.FromArgb(p, v, t);
case 3: return Color.FromArgb(p, q, v);
case 4: return Color.FromArgb(t, p, v);
case 5: return Color.FromArgb(v, p, q);
default: return Color.Black ;
}
}
// Переопределение метода OnPaint для правильной отрисовки
protected override void OnPaint(PaintEventArgs e)
{
base.OnPaint(e);
// Здесь можно добавить дополнительный код для кастомной отрисовки
}
}
}
Проверьте на ошибки, выберу ответ лучшим и запустите как он работает просто у меня ошибка одна пишет
using System;
using System.Drawing;
using System.Windows.Forms;
namespace furrysad
{
static class Program
{
[STAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
Application.Run(new Form1());
}
}
public partial class Form1 : Form
{
private Timer timer;
private float hue = 0f;
private Label label;
public Form1()
{
this.Text = "HackerCom";
this.Size = new Size(800, 600);
this.FormBorderStyle = FormBorderStyle.None;
this.StartPosition = FormStartPosition.CenterScreen;
this.BackColor = Color.Black;
label = new Label();
label.Text = "HACKERCOM";
label.Font = new Font("Arial", 48, FontStyle.Bold);
label.ForeColor = Color.White;
label.AutoSize = true;
label.Location = new Point(this.ClientSize.Width / 2 - label.Width / 2,
this.ClientSize.Height / 2 - label.Height / 2);
label.TextAlign = ContentAlignment.MiddleCenter;
this.Controls.Add(label);
timer = new Timer();
timer.Interval = 30;
timer.Tick += Timer_Tick;
timer.Start();
// Добавляем обработчик клавиши Escape для закрытия формы
this.KeyPreview = true;
this.KeyDown += (s, e) => { if (e.KeyCode == Keys.Escape) this.Close(); };
}
private void Timer_Tick(object sender, EventArgs e)
{
hue += 0.005f;
if (hue > 1) hue = 0;
this.BackColor = ColorFromHSV(hue, 1, 1);
label.ForeColor = Color.FromArgb(255,
(int)(Math.Sin(Environment.TickCount * 0.01) * 127 + 128), 255, 255);
label.Location = new Point(this.ClientSize.Width / 2 - label.Width / 2,
this.ClientSize.Height / 2 - label.Height / 2);
}
public static Color ColorFromHSV(float hue, float saturation, float value)
{
int h = (int)(hue * 360);
int s = (int)(saturation * 255);
int v = (int)(value * 255);
int hi = (int)(h / 60) % 6;
float f = (h / 60f) - (int)(h / 60);
int p = (int)(v * (1 - s / 255f));
int q = (int)(v * (1 - f * s / 255f));
int t = (int)(v * (1 - (1 - f) * s / 255f));
switch (hi)
{
case 0: return Color.FromArgb(v, t, p);
case 1: return Color.FromArgb(q, v, p);
case 2: return Color.FromArgb(p, v, t);
case 3: return Color.FromArgb(p, q, v);
case 4: return Color.FromArgb(t, p, v);
case 5: return Color.FromArgb(v, p, q);
default: return Color.Black;
}
}
protected override void OnPaint(PaintEventArgs e)
{
base.OnPaint(e);
}
}
}