Хочу сделать так чтобы можно было вводить ссылку с http/https почему то без он работает нормально.
помогите исправить код чтобы
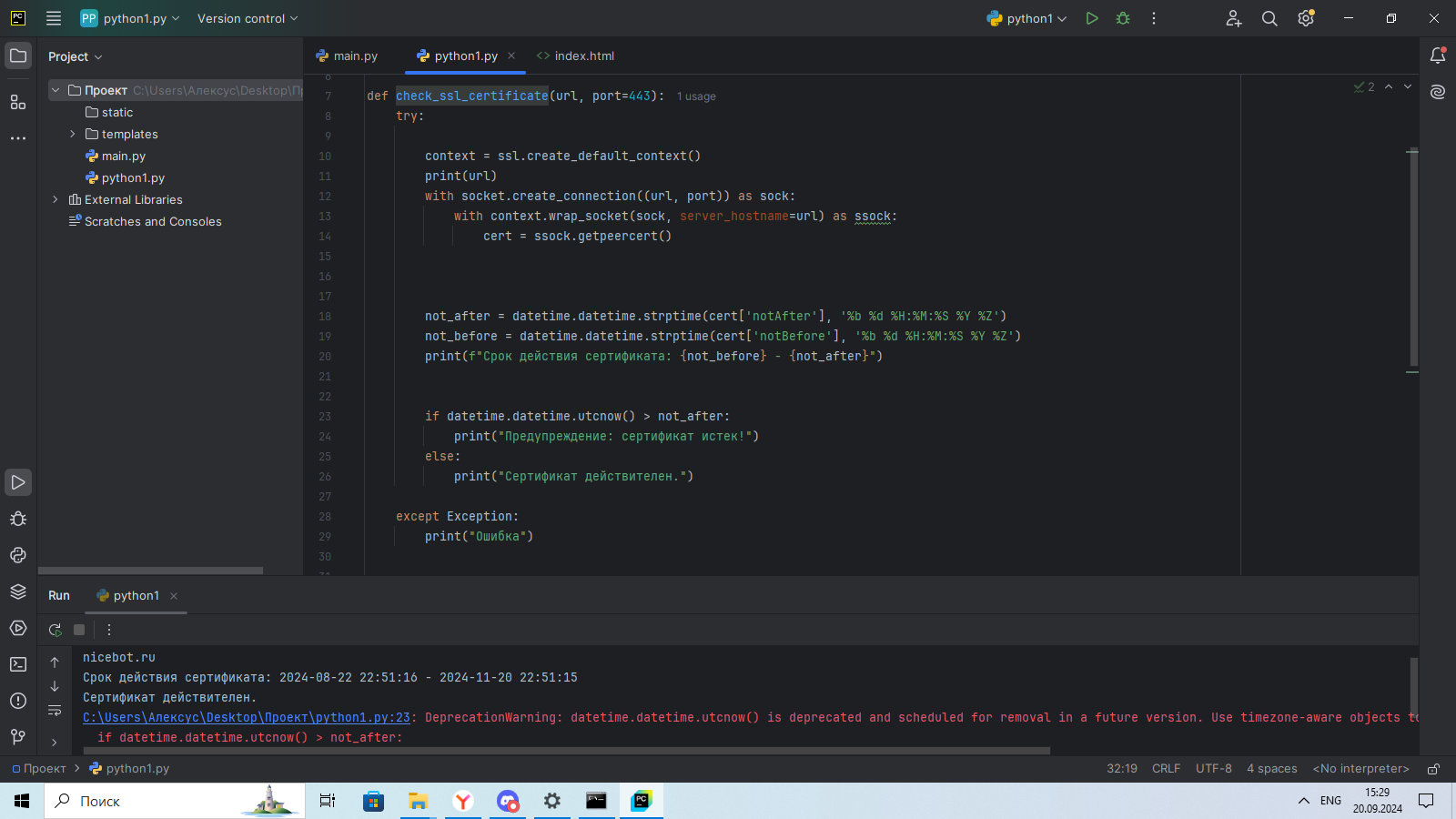
ссылка была с http/https
По дате
По рейтингу
12345678910111213141516171819202122232425262728293031323334353637383940414243444546474849
import ssl
import socket
from datetime import datetime, timezone
from urllib.parse import urlparse
def check_ssl_certificate(url, port=443):
"""Check SSL certificate expiration for a given URL."""
try:
# Parse the URL and extract the hostname
parsed_url = urlparse(url)
hostname = parsed_url.hostname or url
# If scheme (http/https) is missing, assume https
if not parsed_url.scheme:
url = f"https://{url}"
# Create SSL context and connect
context = ssl.create_default_context()
with socket.create_connection((hostname, port)) as sock:
with context.wrap_socket(sock, server_hostname=hostname) as ssock:
cert = ssock.getpeercert()
# Parse the 'notAfter' field from the certificate and make it timezone-aware
not_after = datetime.strptime(
cert['notAfter'], '%b %d %H:%M:%S %Y %Z').replace(tzinfo=timezone.utc)
# Get the current UTC time as timezone-aware
current_time = datetime.now(timezone.utc)
# Check if the certificate is expired or not
expiration_status = (
"Предупреждение: сертификат истек!" if current_time > not_after
else "Сертификат действителен."
)
# Output certificate validity period and status
print(f"Срок действия сертификата: до {not_after}")
print(expiration_status)
except Exception as e:
print(f"Ошибка: {e}")
check_ssl_certificate("example.com")
check_ssl_certificate("google.com")
check_ssl_certificate("globalcd.by")
check_ssl_certificate("mail.ru")
Результат
12345678
Срок действия сертификата: до 2025-03-01 23:59:59+00:00
Сертификат действителен.
Срок действия сертификата: до 2024-11-18 06:33:46+00:00
Сертификат действителен.
Срок действия сертификата: до 2024-11-11 21:06:51+00:00
Сертификат действителен.
Срок действия сертификата: до 2024-10-05 14:10:53+00:00
Сертификат действителен.