SQL(ПРОГРАММИРОВАНИЕ) ОШИБКА ПРИ ЗАПУСКЕ ФОРМЫ
Вообщем написал, ошибок нет, но не запускается.
DAL:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Collections;
using System.Data.SqlClient;
using System.Data.Common;
using System.Configuration;
namespace BankRetail
{
class Dal
{
//stroka soedineniya
string connectionString = @"Data Source=HOME-PC\SQLEXPRESS;Initial Catalog=BANK;Integrated Security=True";
public ArrayList GetAllDebitors()
{
ArrayList allDebitors = new ArrayList();
SqlConnection con = new SqlConnection(connectionString);
SqlCommand com = new SqlCommand("SELECT * FROM Debitors Order by Name", con);
try
{
con.Open();
SqlDataReader dr = com.ExecuteReader();
if (dr.HasRows)
foreach (DbDataRecord result in dr)
allDebitors.Add(result);
}
catch (SqlException ex)
{
//error
}
finally
{
con.Close();
}
return allDebitors;
}
internal object GetAllCreditsForDebitor(string debitorID)
{
ArrayList allCredits = new ArrayList();
using (SqlConnection con = new SqlConnection(connectionString))
{
string query = String.Format("SELECT * FROM Credits Where DebitorID = '{0}' Order By OpenDate ", debitorID);
SqlCommand com = new SqlCommand(query, con);
try
{
con.Open();
SqlDataReader dr = com.ExecuteReader();
if (dr.HasRows)
foreach (DbDataRecord result in dr)
allCredits.Add(result);
}
catch (SqlException ex)
{
//error
}
}
return allCredits;
}
internal ArrayList GetAllPaymentsForCredit(string creditID)
{
ArrayList allPayments = new ArrayList();
using (SqlConnection con = new SqlConnection(connectionString))
{
string query = String.Format("SELECT * FROM Payments Where CreditsID = '{0}' Order By PaymentDate ", creditID);
SqlCommand com = new SqlCommand(query, con);
try
{
con.Open();
SqlDataReader dr = com.ExecuteReader();
if (dr.HasRows)
foreach (DbDataRecord result in dr)
allPayments.Add(result);
}
catch (SqlException ex)
{
//error
}
}
return allPayments;
}
}
}
Ошибка где-то здесь видимо:
//stroka soedineniya
string connectionString = @"Data Source=HOME-PC\SQLEXPRESS;Initial
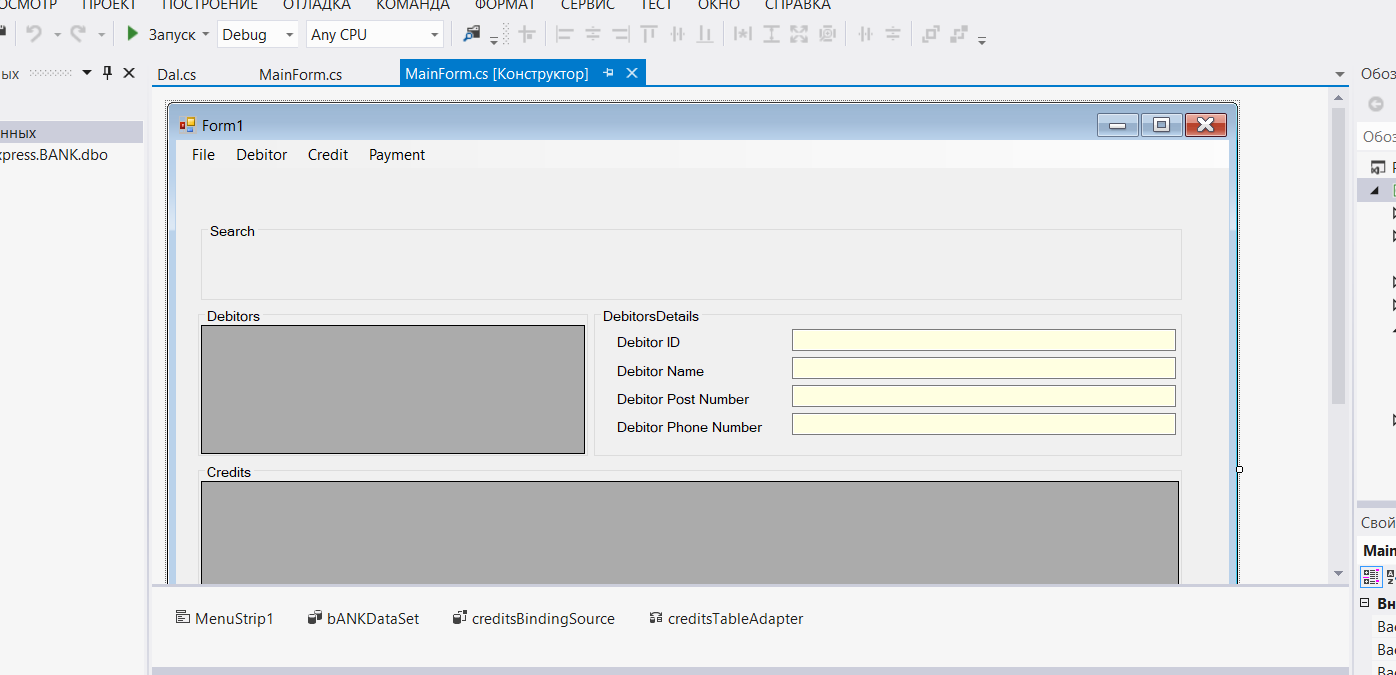
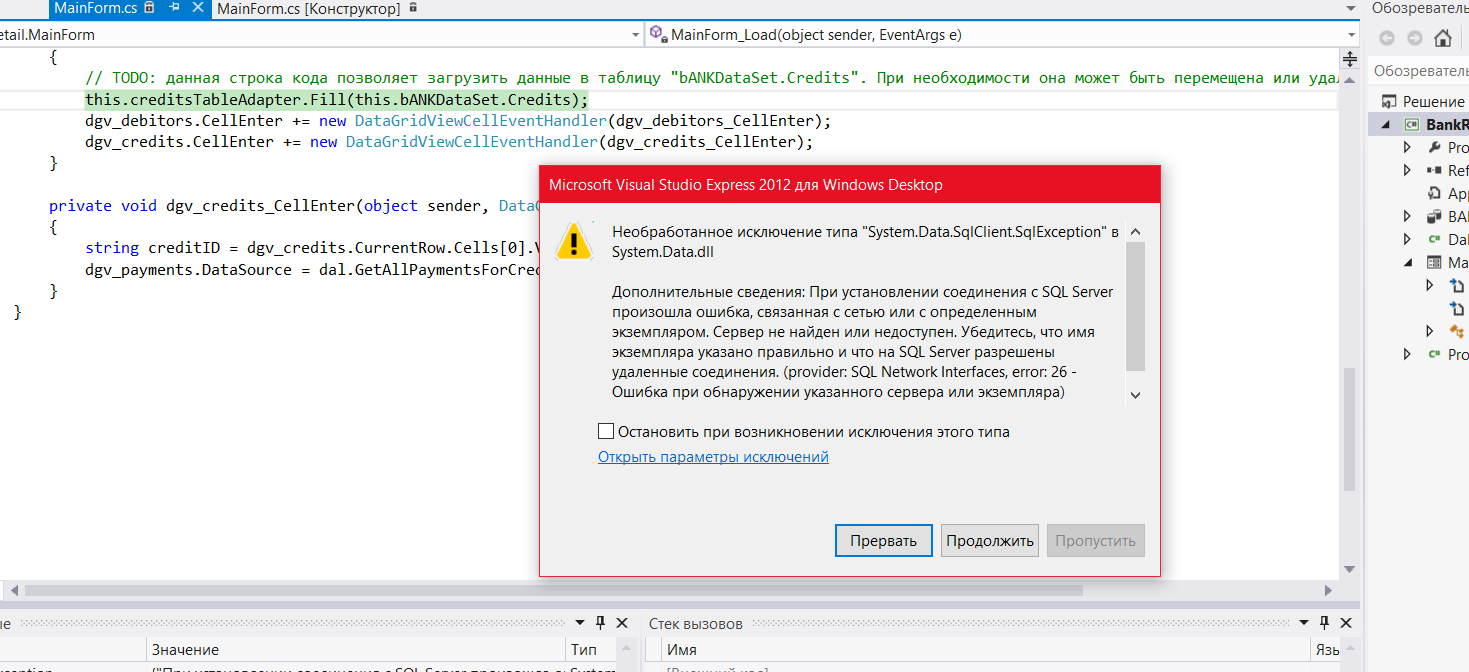
Если БД локальная (на той же машине, на которой запускается код), попробуйте заменить HOME-PC\SQLEXPRESS на .\SQLEXPRESS
Глубже копаться без дополнительных сведений о базе невозможно.