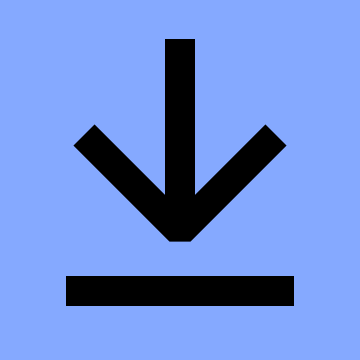
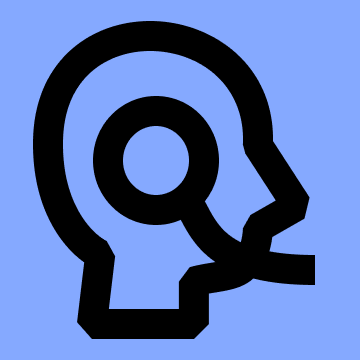
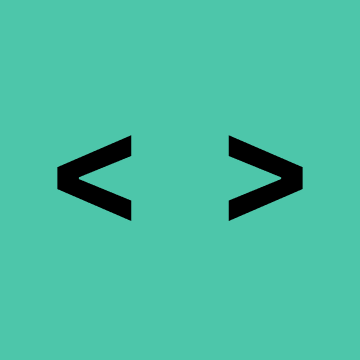
НАПИСАТЬ ПРОГРАММУ НА ЯЗЫКЕ c#
поле шахматной доски определено парой натуральных чисел. УгрАжаит ли полю (a,b) конь, расположенный на поле (c,d), если он делает 2 хода. написать программу на с#
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
// Ввод координат
Console.Write("Введите координаты поля (a, b): ");
var line1 = Console.ReadLine();
var splitLine1 = line1.Split(',');
int a = int.Parse(splitLine1[0]);
int b = int.Parse(splitLine1[1]);
Console.Write("Введите координаты коня (c, d): ");
var line2 = Console.ReadLine();
var splitLine2 = line2.Split(',');
int c = int.Parse(splitLine2[0]);
int d = int.Parse(splitLine2[1]);
bool threatens = DoesKnightThreaten(a, b, c, d);
Console.WriteLine(threatens ? "Да, конь угрожает полю." : "Нет, конь не угрожает полю.");
}
static bool DoesKnightThreaten(int a, int b, int c, int d)
{
// Возможные движения коня
int[,] knightMoves = new int[,]
{
{2, 1}, {2, -1}, {-2, 1}, {-2, -1},
{1, 2}, {1, -2}, {-1, 2}, {-1, -2}
};
// Сначала получаем все возможные позиции после первого хода
HashSet<(int, int)> firstMoves = new HashSet<(int, int)>();
for (int i = 0; i < knightMoves.GetLength(0); i++)
{
int newX = c + knightMoves[i, 0];
int newY = d + knightMoves[i, 1];
// Добавляем только допустимые позиции на доске
if (IsValidPosition(newX, newY))
{
firstMoves.Add((newX, newY));
}
}
// Проверяем, может ли конь из каждой из полученных позиций угрожать (a, b)
foreach (var move in firstMoves)
{
if (CanKnightReach(move.Item1, move.Item2, a, b))
{
return true;
}
}
return false;
}
static bool CanKnightReach(int startX, int startY, int targetX, int targetY)
{
return ((Math.Abs(startX - targetX) == 2 && Math.Abs(startY - targetY) == 1) ||
(Math.Abs(startX - targetX) == 1 && Math.Abs(startY - targetY) == 2));
}
static bool IsValidPosition(int x, int y)
{
// Проверяем, находится ли позиция в пределах шахматной доски 1-8
return x >= 1 && x <= 8 && y >= 1 && y <= 8;
}
}
На
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
Console.WriteLine("♟️ Введите координаты целевого поля (a, b):");
int a = int.Parse(Console.ReadLine());
int b = int.Parse(Console.ReadLine());
Console.WriteLine("🐴 Введите координаты коня (c, d):");
int c = int.Parse(Console.ReadLine());
int d = int.Parse(Console.ReadLine());
Console.WriteLine(IsThreatened(a, b, c, d)
? $"⚠️ Конь угрожает полю ({a}, {b}) через два хода."
: $"✅ Конь не угрожает полю ({a}, {b}) через два хода.");
Console.WriteLine("Спасибо за использование программы! 🎉");
}
static bool IsThreatened(int a, int b, int c, int d)
{
int[,] moves = { { 2, 1 }, { 2, -1 }, { -2, 1 }, { -2, -1 }, { 1, 2 }, { 1, -2 }, { -1, 2 }, { -1, -2 } };
var firstMoves = new HashSet<(int, int)>();
foreach (var move in moves)
{
int newC = c + move[0], newD = d + move[1];
if (IsValidPosition(newC, newD)) firstMoves.Add((newC, newD));
}
foreach (var move in firstMoves)
{
foreach (var secondMove in moves)
{
int newC2 = move.Item1 + secondMove[0], newD2 = move.Item2 + secondMove[1];
if (IsValidPosition(newC2, newD2) && newC2 == a && newD2 == b) return true;
}
}
return false;
}
static bool IsValidPosition(int x, int y) => x >= 1 && x <= 8 && y >= 1 && y <= 8;
}
учи C# если надо писать на C#